
So to sort with “two keys”, you can define a key function that returns a tuple rather than only a single tuple value. Only if there’s a tie, it takes the second tuple value and so on. Per default, Python sorts tuples lexicographically-the first tuple value is considered first. (1,2) and (3,2)), you want to sort after the first tuple value.
#Python sort list how to#
How to sort a list with two keys? For example, you have a list of tuples and you want to sort after the second tuple value first. The itemgetter() function does exactly the same as the lambda function in the previous example: it returns the second tuple value and uses it as a basis for comparison.
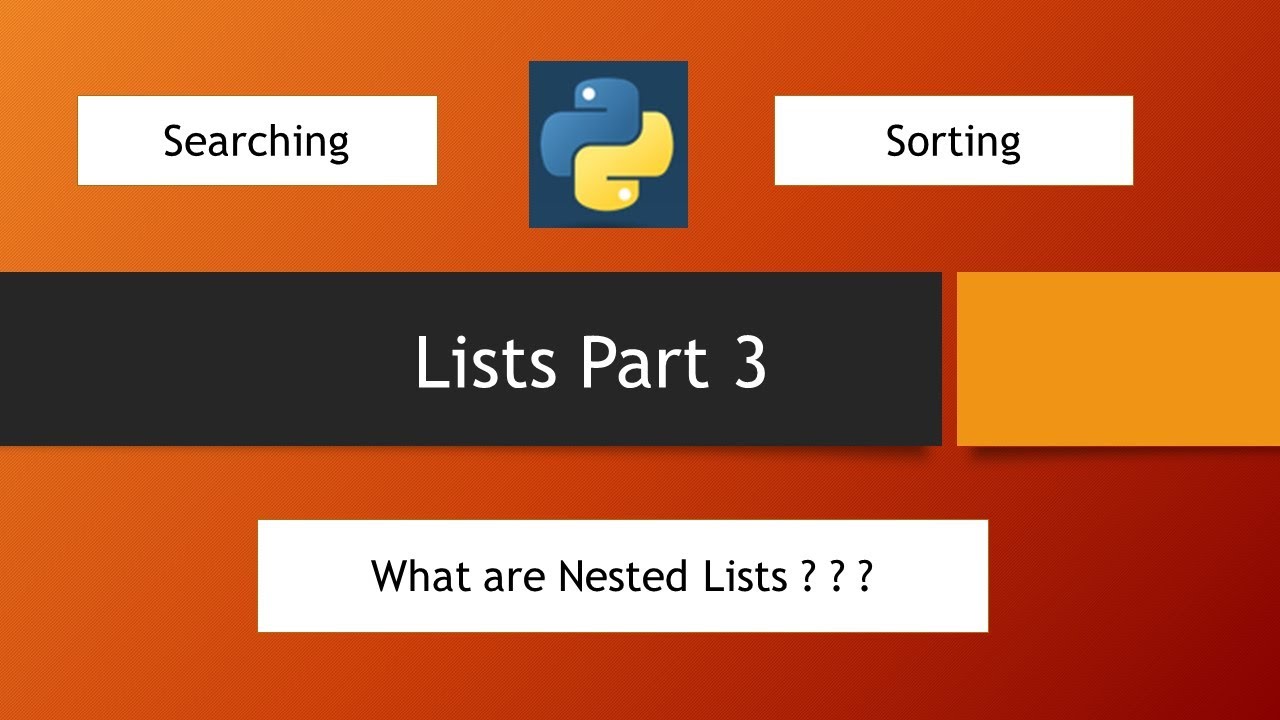
You can use any function as a key function that transforms one element into another (comparable) element.įor example, it’s common to use the itemgetter() function from the operator module to access the i-th value of an iterable: > from operator import itemgetter An Introduction to Lambda Functions in Python.You achieve this by defining a key function key=lambda x: x that takes one list element x (a tuple) as an argument and transforms it into a comparable value x (the second tuple value). In the third example, the list is sorted according to the second tuple value first. You can see that in the first two examples, the list is sorted according to the first tuple value first. Hence, the key function takes one input argument (a list element) and returns one output value (a value that can be compared). The key function is then called on each list element and returns another value based on which the sorting is done. The list.sort() method takes another function as an optional key argument that allows you to modify the default sorting behavior. Why? Because it sorts a list in-place and doesn’t create a new list. Return value: The method list.sort() returns None, no matter the list on which it’s called. Default False.) The order of the list elements. Then, the method sorts based on the key function results rather than the elements themselves. The function is then applied to each element in the list. Default None.) Pass a function that takes a single argument and returns a comparable value. Syntax: You can call this method on each list object in Python ( Python versions 2.x and 3.x). You can also solve this puzzle and track your Python skills on our interactive Finxter app.
#Python sort list code#
# What's the output of this code snippet? Let’s deepen your understanding with a short code puzzle-can you solve it? # Create an unsorted integer list You then reverse the order of the elements in the sorted list using the reverse=True argument. You then sort the list once using the default sorting behavior and once using a customized sorting behavior with only the first letter of the number. In the first line of the example, you create the list lst.

Here’s a short overview example that shows you how to use the arguments in practice: # Create an unsorted integer list
